mirror of
https://github.com/esp8266/Arduino.git
synced 2025-06-07 16:23:38 +03:00
Merge remote-tracking branch 'remotes/esp8266/master' into httpClient
This commit is contained in:
commit
b845d03b89
@ -25,14 +25,10 @@
|
||||
|
||||
size_t StreamString::write(const uint8_t *buffer, size_t size) {
|
||||
if(reserve(length() + size + 1)) {
|
||||
for(size_t i = 0; i < size; i++) {
|
||||
if(write(*buffer)) {
|
||||
buffer++;
|
||||
} else {
|
||||
return i;
|
||||
}
|
||||
}
|
||||
|
||||
const uint8_t *s = buffer;
|
||||
const uint8_t *end = buffer + size;
|
||||
while(write(*s++) && s < end);
|
||||
return s - buffer;
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
|
@ -60,6 +60,10 @@ void cont_yield(cont_t*);
|
||||
// return 1 if guard bytes were overwritten.
|
||||
int cont_check(cont_t* cont);
|
||||
|
||||
// Go through stack and check how many bytes are most probably still unchanged
|
||||
// and thus weren't used by the user code. i.e. that stack space is free. (high water mark)
|
||||
int cont_get_free_stack(cont_t* cont);
|
||||
|
||||
// Check if yield() may be called. Returns true if we are running inside
|
||||
// continuation stack
|
||||
bool cont_can_yield(cont_t* cont);
|
||||
|
@ -30,6 +30,12 @@ void ICACHE_RAM_ATTR cont_init(cont_t* cont) {
|
||||
cont->stack_guard2 = CONT_STACKGUARD;
|
||||
cont->stack_end = cont->stack + (sizeof(cont->stack) / 4);
|
||||
cont->struct_start = (unsigned*) cont;
|
||||
|
||||
// fill stack with magic values to check high water mark
|
||||
for(int pos = 0; pos < sizeof(cont->stack) / 4; pos++)
|
||||
{
|
||||
cont->stack[pos] = CONT_STACKGUARD;
|
||||
}
|
||||
}
|
||||
|
||||
int ICACHE_RAM_ATTR cont_check(cont_t* cont) {
|
||||
@ -38,6 +44,19 @@ int ICACHE_RAM_ATTR cont_check(cont_t* cont) {
|
||||
return 0;
|
||||
}
|
||||
|
||||
int ICACHE_RAM_ATTR cont_get_free_stack(cont_t* cont) {
|
||||
uint32_t *head = cont->stack;
|
||||
int freeWords = 0;
|
||||
|
||||
while(*head == CONT_STACKGUARD)
|
||||
{
|
||||
head++;
|
||||
freeWords++;
|
||||
}
|
||||
|
||||
return freeWords * 4;
|
||||
}
|
||||
|
||||
bool ICACHE_RAM_ATTR cont_can_yield(cont_t* cont) {
|
||||
return !ETS_INTR_WITHINISR() &&
|
||||
cont->pc_ret != 0 && cont->pc_yield == 0;
|
||||
|
@ -66,11 +66,11 @@ public:
|
||||
|
||||
bool rename(const char* pathFrom, const char* pathTo) override {
|
||||
if (!isSpiffsFilenameValid(pathFrom)) {
|
||||
DEBUGV("SPIFFSImpl::rename: invalid pathFrom=`%s`\r\n", path);
|
||||
DEBUGV("SPIFFSImpl::rename: invalid pathFrom=`%s`\r\n", pathFrom);
|
||||
return false;
|
||||
}
|
||||
if (!isSpiffsFilenameValid(pathTo)) {
|
||||
DEBUGV("SPIFFSImpl::rename: invalid pathTo=`%s` \r\n", path);
|
||||
DEBUGV("SPIFFSImpl::rename: invalid pathTo=`%s` \r\n", pathTo);
|
||||
return false;
|
||||
}
|
||||
auto rc = SPIFFS_rename(&_fs, pathFrom, pathTo);
|
||||
|
@ -71,5 +71,8 @@ platformio run --target upload
|
||||
- [IDE Integration](http://docs.platformio.org/en/latest/ide.html) (Atom, CLion, Eclipse, Qt Creator, Sublime Text, VIM, Visual Studio)
|
||||
- [Project Examples](http://docs.platformio.org/en/latest/platforms/espressif.html#examples)
|
||||
|
||||
## Demo of OTA update
|
||||
[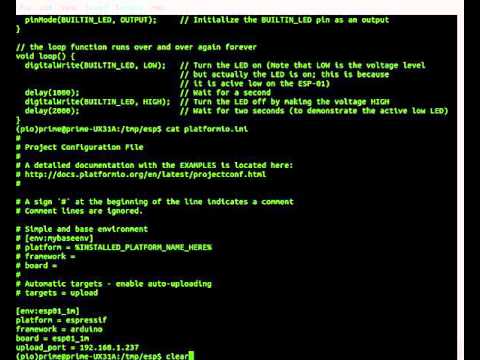](http://www.youtube.com/watch?v=W8wWjvQ8ZQs "PlatformIO and OTA firmware uploading to Espressif ESP8266 ESP-01")
|
||||
## Demo of Over-the-Air (OTA) ESP8266 programming using PlatformIO
|
||||
|
||||
http://www.penninkhof.com/2015/12/1610-over-the-air-esp8266-programming-using-platformio/
|
||||
|
||||
[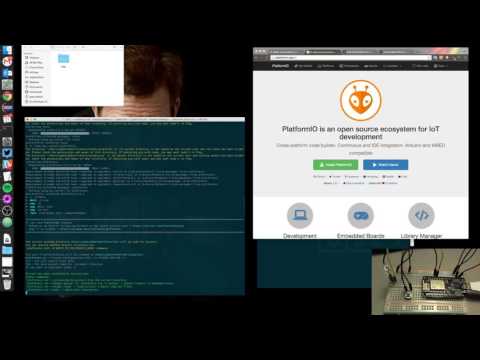](http://www.youtube.com/watch?v=lXchL3hpDO4 "Over-the-Air ESP8266 programming using PlatformIO")
|
||||
|
@ -367,7 +367,12 @@ int HTTPClient::sendRequest(const char * type, Stream * stream, size_t size) {
|
||||
int c = stream->readBytes(buff, ((s > buff_size) ? buff_size : s));
|
||||
|
||||
// write it to Stream
|
||||
bytesWritten += _tcp->write((const uint8_t *) buff, c);
|
||||
int w = _tcp->write((const uint8_t *) buff, c);
|
||||
if(w != c) {
|
||||
DEBUG_HTTPCLIENT("[HTTP-Client][sendRequest] short write asked for %d but got %d\n", c, w);
|
||||
break;
|
||||
}
|
||||
bytesWritten += c;
|
||||
|
||||
if(len > 0) {
|
||||
len -= c;
|
||||
@ -382,7 +387,7 @@ int HTTPClient::sendRequest(const char * type, Stream * stream, size_t size) {
|
||||
free(buff);
|
||||
|
||||
if(size && (int) size != bytesWritten) {
|
||||
DEBUG_HTTPCLIENT("[HTTP-Client][sendRequest] Stream payload bytesWritten %d and size %d mismatch!.\n", bytesWritten, _size);
|
||||
DEBUG_HTTPCLIENT("[HTTP-Client][sendRequest] Stream payload bytesWritten %d and size %d mismatch!.\n", bytesWritten, size);
|
||||
DEBUG_HTTPCLIENT("[HTTP-Client][sendRequest] ERROR SEND PAYLOAD FAILED!");
|
||||
return HTTPC_ERROR_SEND_PAYLOAD_FAILED;
|
||||
} else {
|
||||
@ -390,7 +395,7 @@ int HTTPClient::sendRequest(const char * type, Stream * stream, size_t size) {
|
||||
}
|
||||
|
||||
} else {
|
||||
DEBUG_HTTPCLIENT("[HTTP-Client][writeToStream] too less ram! need " HTTP_TCP_BUFFER_SIZE);
|
||||
DEBUG_HTTPCLIENT("[HTTP-Client][sendRequest] too less ram! need %d\n", HTTP_TCP_BUFFER_SIZE);
|
||||
return HTTPC_ERROR_TOO_LESS_RAM;
|
||||
}
|
||||
|
||||
@ -474,7 +479,12 @@ int HTTPClient::writeToStream(Stream * stream) {
|
||||
int c = _tcp->readBytes(buff, ((size > buff_size) ? buff_size : size));
|
||||
|
||||
// write it to Stream
|
||||
bytesWritten += stream->write(buff, c);
|
||||
int w = stream->write(buff, c);
|
||||
if(w != c) {
|
||||
DEBUG_HTTPCLIENT("[HTTP-Client][writeToStream] short write asked for %d but got %d\n", c, w);
|
||||
break;
|
||||
}
|
||||
bytesWritten += c;
|
||||
|
||||
if(len > 0) {
|
||||
len -= c;
|
||||
@ -495,7 +505,7 @@ int HTTPClient::writeToStream(Stream * stream) {
|
||||
}
|
||||
|
||||
} else {
|
||||
DEBUG_HTTPCLIENT("[HTTP-Client][writeToStream] too less ram! need " HTTP_TCP_BUFFER_SIZE);
|
||||
DEBUG_HTTPCLIENT("[HTTP-Client][writeToStream] too less ram! need %d\n", HTTP_TCP_BUFFER_SIZE);
|
||||
return HTTPC_ERROR_TOO_LESS_RAM;
|
||||
}
|
||||
|
||||
|
@ -3,6 +3,7 @@
|
||||
|
||||
class RequestHandler {
|
||||
public:
|
||||
virtual ~RequestHandler() { }
|
||||
virtual bool canHandle(HTTPMethod method, String uri) { return false; }
|
||||
virtual bool canUpload(String uri) { return false; }
|
||||
virtual bool handle(ESP8266WebServer& server, HTTPMethod requestMethod, String requestUri) { return false; }
|
||||
|
@ -68,7 +68,7 @@ enum wl_enc_type { /* Values map to 802.11 encryption suites... */
|
||||
ENC_TYPE_AUTO = 8
|
||||
};
|
||||
|
||||
#if !defined(LWIP_INTERNAL)
|
||||
#if !defined(LWIP_INTERNAL) && !defined(__LWIP_TCP_H__)
|
||||
enum wl_tcp_state {
|
||||
CLOSED = 0,
|
||||
LISTEN = 1,
|
||||
|
@ -299,7 +299,7 @@ static void ATTR_GDBFN sendReason() {
|
||||
} else if (gdbstub_savedRegs.reason&0x80) {
|
||||
//We stopped because of an exception. Convert exception code to a signal number and send it.
|
||||
i=gdbstub_savedRegs.reason&0x7f;
|
||||
if (i<sizeof(exceptionSignal)) return gdbPacketHex(exceptionSignal[i], 8); else gdbPacketHex(11, 8);
|
||||
if (i<sizeof(exceptionSignal)) gdbPacketHex(exceptionSignal[i], 8); else gdbPacketHex(11, 8);
|
||||
} else {
|
||||
//We stopped because of a debugging exception.
|
||||
gdbPacketHex(5, 8); //sigtrap
|
||||
|
Binary file not shown.
Loading…
x
Reference in New Issue
Block a user